Matlab Matrices
Lately, I have been taking the amazing and very famous Andrews NG Machine Learning Classes on Coursera platform!!
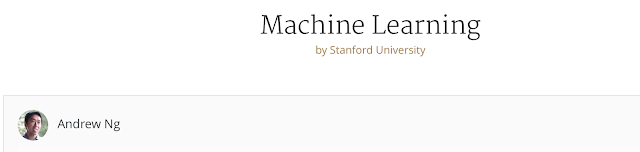
I am amazed on how everything is presented easily and simplified. The exercises and practical assignments come with a lot of code in tutorial code that we need to fill in the gaps.
My conclusion is that in order to master Matlab you have first to master Matlab matrices and vectors. If you want speed in calculation we have first to "matrixize" formulas.
Following are some of the best and most used tips on matrixes (from Quora question).
Always useful is Mathworks help article.
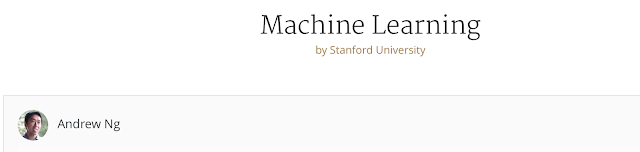
I am amazed on how everything is presented easily and simplified. The exercises and practical assignments come with a lot of code in tutorial code that we need to fill in the gaps.
My conclusion is that in order to master Matlab you have first to master Matlab matrices and vectors. If you want speed in calculation we have first to "matrixize" formulas.
Following are some of the best and most used tips on matrixes (from Quora question).
Always useful is Mathworks help article.
x = zeros(n,m); % initialize x to all zeros
x = ones(n,m) * 5; % initialize all x to 5s
x(4,:) = 5; % assign 5 to all elements of row #4
x(:,1) = x(:,1) + x(:,2);% add column #2 to column #1
y = x(2:5, 1:6); % copy inner block rows 2-5 and columns 1-6 to y
z = x + y; % add all elements of x and y, store in z
z = x * y; % matrix multiply x and y, store in z
z = x .* y; % individually multiply elements of x and y, store in z
z = x .^ 2; % set each element of z to the same element of x squared
w = find(x == 5); % search x for elements == 5, store integer indices in w
w = (x == 5); % create binary matrix w that has a 1 anywhere x == 5
x(w) = 8; % set value of all elements found above (either case) to 8
z = sum(x(:) < 1); % count how many elements have value < 1
x(x < 0) = 0; % set all negative elements of x to zero
y = mean(x, 1); % set y(1,i) to the average of each column of x
y = mean(x(:)); % set y to the average of all elements of x
y = mean2(x); % set y to the average of all elements of x (2D only)
x = [y, x]; % prepend columns of y to matrix x
x(:,end+1) = 5; % add a column of 5s to the right side of x
z = sqrt(mean(x(:).^2)); % set z to the root-mean-square of all elements in x
if any(x(:) == 5) % true if any element of x == 5
if all(x(:) == 0) % true if all elements of x == 0
if any(all(x == 0, 2)) % true if any rows are all zeros
x = bsxfun(@times, x, 1./sum(x)); % normalize x so each column sums to 1
x = (x - min(x(:))) ./ (max(x(:)) - min(x(:))); % normalize x so min==0 and max==1
Σχόλια